Extracting XML documents from relational databases bridges the gap between structured, tabular data storage and the hierarchical, flexible nature of XML. This comprehensive guide combines conceptual insights with practical methods, offering an in-depth understanding of how to efficiently transform relational data into XML for diverse applications.
Introduction to Extracting XML Documents from Relational Databases
Relational databases store data in a structured format of rows and columns. While this format excels at operational tasks, XML offers distinct advantages for data exchange, hierarchical representation, and integration with other systems. Extracting XML documents allows you to:
- Share data via APIs or web services.
- Represent nested data relationships effectively.
- Migrate or integrate data across platforms.
Table of Contents
Two main approaches dominate XML extraction: database mapping and native XML support.
Approaches to XML Extraction
Database Mapping
This approach maps relational database fields to XML elements. It dynamically converts SQL query results into XML documents, often using server-side web applications or specialized tools.
- Concept: Relational data remains in the database while XML conversion occurs at runtime.
- Tools: Many systems provide configuration files or graphical tools to define mappings.
Use this ER schema diagram for a simplified UNIVERSITY database.
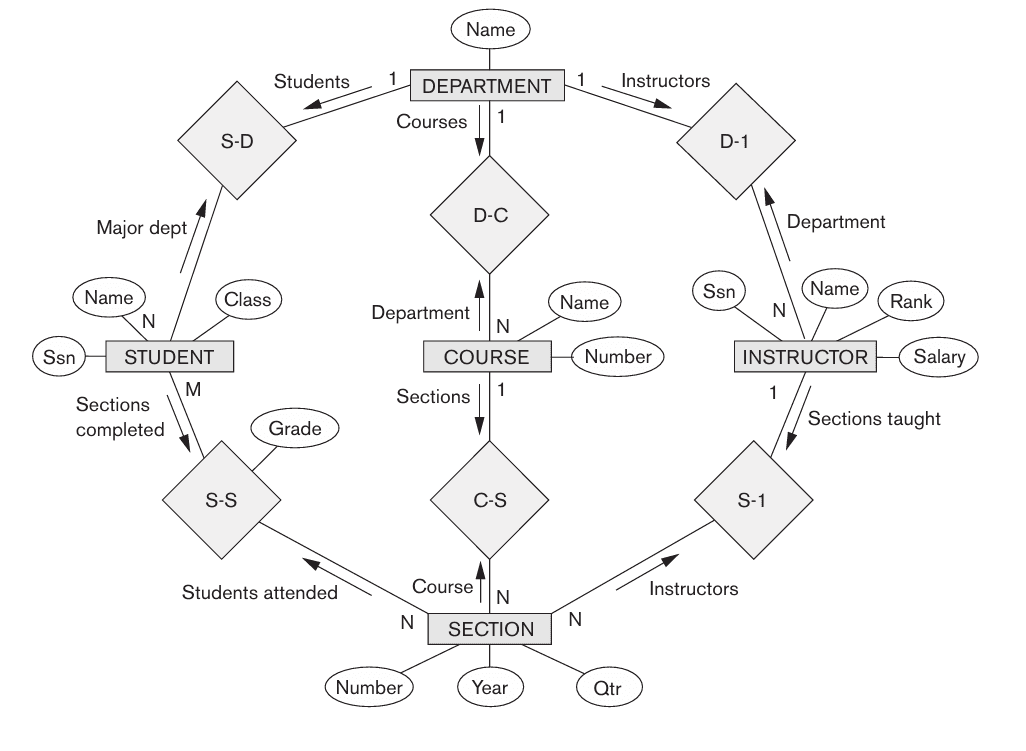
Example tools for database mapping include Talend and DBMS-specific utilities like SQL Server’s FOR XML clause or Oracle’s DBMS_XMLGEN package.
Native XML Support
Some databases store data as XML natively, rather than converting it from relational structures. These systems serialize XML data into a clean format for storage and retrieval.
- Concept: Data is stored and retrieved directly as XML documents.
- Use Case: Best suited for applications with XML-centric workflows.
Examples of products supporting native XML include Oracle XML DB and MarkLogic.
Practical Methods for XML Extraction
Using SQL/XML Functions
SQL Server Example
SQL Server’s FOR XML clause generates XML directly from query results:
SELECT
EmployeeID,
FirstName,
LastName
FROM
Employees
FOR XML AUTO;
- AUTO: Generates nested XML based on query structure.
- RAW: Produces flat, row-based XML.
- PATH: Offers granular control over XML formatting.
Oracle Example
Oracle provides the DBMS_XMLGEN package for dynamic XML generation:
SELECT
DBMS_XMLGEN.getXML('SELECT EmployeeID, FirstName, LastName FROM Employees')
AS XML_DATA
FROM dual;
Oracle DBMS_XMLGEN Utility
This utility generates XML directly from SQL queries, ideal for Oracle users.
DECLARE
ctxHandle DBMS_XMLGEN.ctxHandle;
BEGIN
ctxHandle := DBMS_XMLGEN.newContext('SELECT * FROM employees');
DBMS_XMLGEN.getXML(ctxHandle);
DBMS_XMLGEN.closeContext(ctxHandle);
END;
Using Scripting Languages
Languages like Python or Java can extract data from relational databases and transform it into XML. Here’s an example using Python:
import xml.etree.ElementTree as ET
import sqlite3
# Connect to the database
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
# Query data
cursor.execute("SELECT EmployeeID, FirstName, LastName FROM Employees")
rows = cursor.fetchall()
# Create XML structure
root = ET.Element("Employees")
for row in rows:
employee = ET.SubElement(root, "Employee")
ET.SubElement(employee, "EmployeeID").text = str(row[0])
ET.SubElement(employee, "FirstName").text = row[1]
ET.SubElement(employee, "LastName").text = row[2]
# Write to XML file
tree = ET.ElementTree(root)
tree.write("employees.xml")
Using ETL Tools
ETL tools like Talend and Informatica streamline XML extraction, especially for large-scale data pipelines.
Challenges in XML Extraction
- Complex Relationships: Mapping normalized relational tables into hierarchical XML structures can be challenging.
- Performance Overhead: Real-time XML generation can slow down database operations.
- Data Volume: Large datasets can result in bulky XML files, increasing processing time.
Best Practices
- Optimize Queries: Use database indexing and efficient SQL constructs to minimize overhead.
- Use Schema Validation: Ensure XML adheres to a defined schema (e.g., XSD) for consistency.
- Handle Large Data with Pagination: Extract data in smaller batches to avoid memory overload.
- Leverage Native Features: Where available, use DBMS-native XML tools for efficiency.
Applications of XML Extraction
- Web Services: XML is widely used in SOAP-based services and certain REST APIs.
- Data Migration: Acts as an intermediate format during database migrations.
- Configuration Files: Many applications use XML for configuration management.
- Report Generation: XML’s hierarchical format is well-suited for generating complex reports.
Conclusion
By combining theoretical insights with practical implementations, this guide equips you to extract XML documents efficiently from relational databases. Whether you’re leveraging SQL functions, scripting languages, or ETL tools, the key is to choose an approach that aligns with your use case and data complexity.