When working with REST Assured for API testing, you often need to handle JSON or XML request and response payloads. One of the best ways to manage these payloads is by using POJO classes. But what is POJO class in REST Assured, and how does it help in API automation?
In this blog post, we’ll break down POJO in REST Assured in simple terms, explain its benefits, and show how to use it effectively.
What is POJO Class?
POJO stands for Plain Old Java Object. It is a simple Java class with private fields, getters, setters, and a default constructor. A POJO class in REST Assured is used to represent JSON or XML request/response structures in an object-oriented way.
Why Use POJO in REST Assured?
- Clean Code: Instead of dealing with raw JSON strings, you work with Java objects.
- Reusability: The same POJO class can be reused across multiple API tests.
- Easy Maintenance: If the API structure changes, you only update the POJO class instead of multiple test scripts.
- Better Readability: Code becomes more structured and easier to understand.
Example of a POJO Class in REST Assured
Let’s say we have a JSON payload for a user:
{
"name": "John Doe",
"age": 30,
"email": "john.doe@example.com"
}
To represent this in a POJO class, we create a Java class like this:
public class User {
// Private fields
private String name;
private int age;
private String email;
// Default constructor (required for JSON serialization/deserialization)
public User() {
}
// Parameterized constructor (optional)
public User(String name, int age, String email) {
this.name = name;
this.age = age;
this.email = email;
}
// Getters and Setters
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
How to Use POJO in REST Assured?
1. Sending a POST Request with POJO
Instead of sending a raw JSON string, we can use the POJO class to create the request body:
import io.restassured.RestAssured;
import io.restassured.http.ContentType;
public class TestAPI {
public static void main(String[] args) {
// Create a User POJO object
User user = new User();
user.setName("John Doe");
user.setAge(30);
user.setEmail("john.doe@example.com");
// Send POST request using REST Assured
RestAssured.given()
.contentType(ContentType.JSON)
.body(user) // Pass the POJO object directly
.post("https://api.example.com/users")
.then()
.statusCode(201);
}
}
2. Converting JSON Response to POJO
If the API returns a JSON response, we can map it back to a POJO class:
import io.restassured.RestAssured;
import io.restassured.response.Response;
public class TestAPI {
public static void main(String[] args) {
// Send GET request and convert response to User POJO
User user = RestAssured.given()
.get("https://api.example.com/users/1")
.as(User.class); // Convert JSON response to User object
System.out.println("Name: " + user.getName());
System.out.println("Age: " + user.getAge());
System.out.println("Email: " + user.getEmail());
}
}
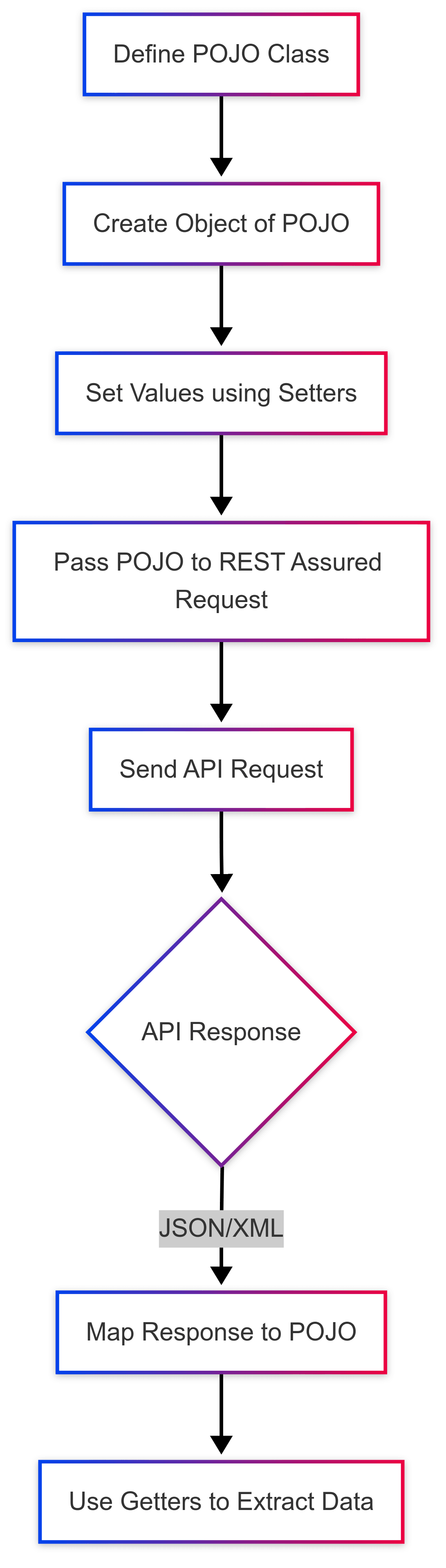
- Define POJO Class
- Create a Java class with fields matching the API’s JSON/XML structure (e.g., User.java).
- Includes private fields, getters, setters, and constructors.
- Create POJO Object
- Instantiate the class: User user = new User();.
- Set Values
- Populate data using setters
- Pass POJO to REST Assured
- Use the POJO directly in the request body
.body(user) // No manual JSON!
- Send API Request
- Execute the request (e.g., POST, GET).
- Handle Response
- If the API returns JSON/XML, map it back to the POJO
User responseUser = response.as(User.class);
- Extract Data
- Use getters to validate or print values
Key Takeaways from the Flowchart
- Eliminates Manual JSON Handling: No more String jsonBody = “{\”name\”:\”John\”}”;.
- Two-Way Conversion: POJOs work for both requests (Java → JSON) and responses (JSON → Java).
- Centralized Control: Change the POJO once if the API schema updates.
Advantages of Using POJO in REST Assured
- Structured Data Handling: Instead of parsing JSON manually, POJO classes handle it automatically.
- Type Safety: Java ensures data types are correct (e.g., age is an int, not a string).
- Easy Modifications: If the API schema changes, you update only the POJO class.
- Supports Nested JSON: You can create nested POJO classes for complex JSON structures.
Conclusion
Using a POJO class in REST Assured makes API testing cleaner, more maintainable, and easier to read. Instead of dealing with raw JSON, you work with Java objects, improving code reusability and reducing errors.
If you’re automating API tests with REST Assured, start using POJO classes today for better-structured and more efficient test scripts!
Final Thoughts
- POJO in REST Assured simplifies JSON/XML handling.
- It improves code readability and reusability.
- Always use getters and setters for proper serialization.
By now, you should have a clear understanding of what is a POJO class in REST Assured and how to use it effectively. Happy testing! 🚀