When developing applications, understanding the concept of a method signature in Java is important. It uniquely identifies a method within a class and plays a major role in features like method overloading. In this blog, we will dive into the components, explore common misconceptions, and provide practical examples to clarify its usage.
What is Method Signature in Java?
In Java, a method signature is defined by two primary components:
- Method Name: The name of the method, which should be a valid identifier and follow Java naming conventions.
- Parameter List: The sequence and types of parameters in the method’s parentheses. This includes:
- The number of parameters
- The data types of parameters
- The order of parameters
This concept does not include the return type, access modifiers, or other method modifiers like static or final.
Importance of Method Signature in Java
- Method Overloading: Differentiating between methods with the same name but different parameter lists.
- Compile-Time Checking: The compiler uses this concept to resolve method calls and ensure correctness.
- Code Clarity and Design: A well-defined method signature promotes better readability and maintainability.
Examples:
class Example {
// Method 1: No parameters
void display() {
System.out.println("No parameters");
}
// Method 2: One integer parameter
void display(int num) {
System.out.println("Integer: " + num);
}
// Method 3: Two parameters of different types
void display(String message, double value) {
System.out.println("Message: " + message + ", Value: " + value);
}
public static void main(String[] args) {
Example obj = new Example();
// Calls Method 1
obj.display();
// Calls Method 2
obj.display(5);
// Calls Method 3
obj.display("Hello", 3.14);
}
}
Explanation:
- display(): No parameters → Signature: display()
- display(int num): Single parameter of type int → Signature: display(int)
- display(String message, double value): Two parameters → Signature: display(String, double)
The method name is the same (display), but the parameter list is different, making each signature unique.
Key Points
- Overloading depends on unique method signatures.
- Changing only the return type does not create a unique signature. For example:
void display() {}
int display() {} // Compilation error
- This ensures clarity and avoids ambiguity in method calls.
Here’s a deeper dive into method signatures in Java with additional aspects that can enrich your understanding:
Method Overriding and Signatures
What is Method Overriding?
Method overriding occurs when a subclass provides its own implementation of a method that is already defined in its superclass. For the override to work:
→ Flow Chart for better understanding
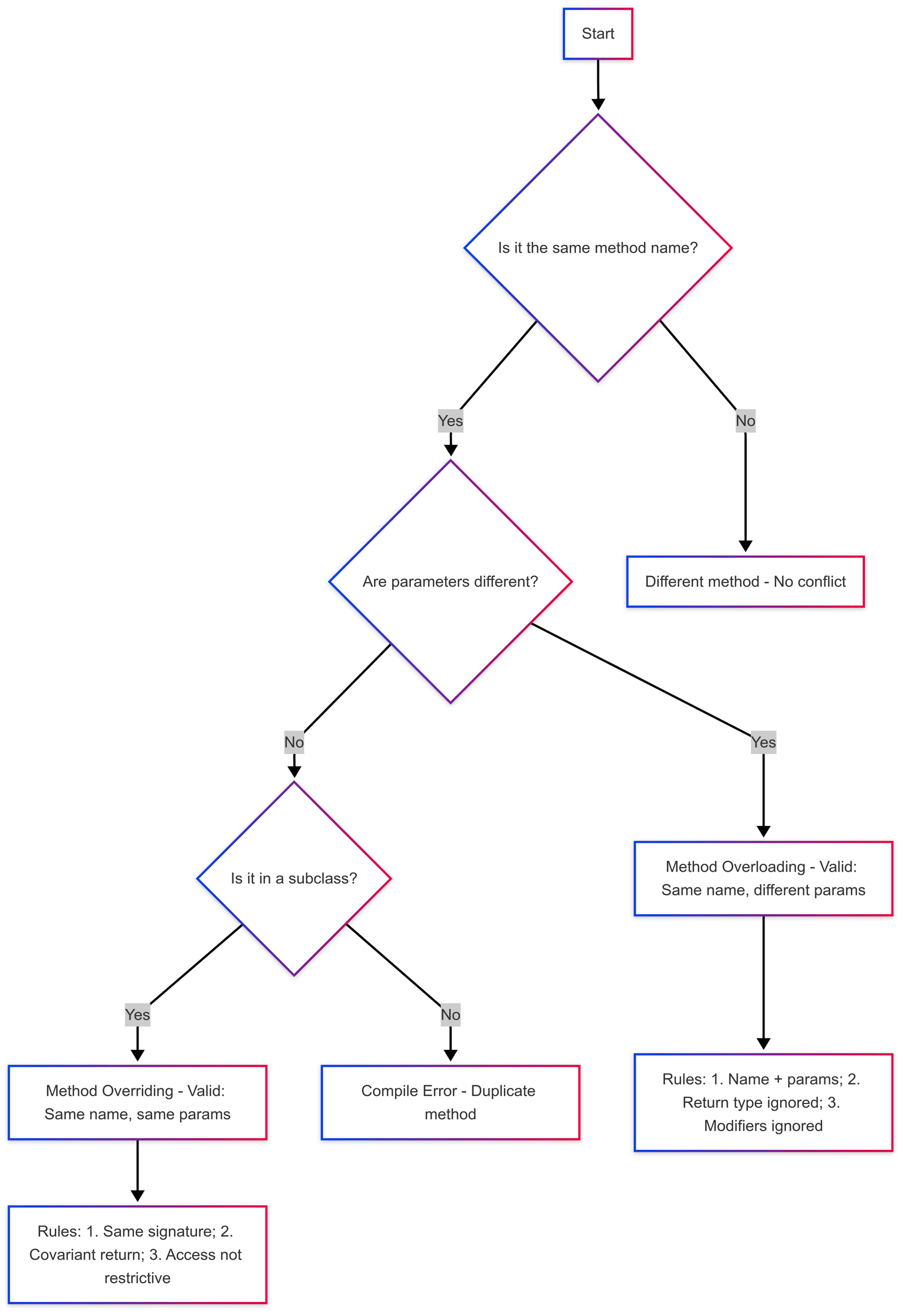
- The method signature (name + parameter list) must exactly match the parent class method.
- The return type should be the same (or a covariant subtype, e.g., returning Dog instead of Animal).
- Access modifiers cannot be more restrictive (e.g., overriding a protected method with public is allowed, but not vice versa).
Example:
class Animal {
void makeSound() { // Original method
System.out.println("Animal sound");
}
}
class Dog extends Animal {
@Override
void makeSound() { // Overridden method (same signature)
System.out.println("Bark!");
}
}
Key Point:
Unlike overloading, overriding depends on inheritance and requires signature consistency.
Advanced Topics: Varargs and Generics
→ Varargs in Method Signature
Varargs (variable arguments) allow a method to accept any number of values of the same type. They are treated as arrays in the signature.
Example:
void printNumbers(int... numbers) { // Signature: printNumbers(int[])
for (int num : numbers) {
System.out.println(num);
}
}
Note:
- Varargs (…) must be the last parameter in a method.
- Overloading with varargs can be tricky (e.g., printNumbers(int) vs. printNumbers(int…) may cause ambiguity).
→ Generic Methods
Generic methods use type parameters (e.g., <T>) to work with different data types while keeping the same signature structure.
Example:
void displayList(List list) { // Signature: displayList(List)
for (T item : list) {
System.out.println(item);
}
}
Key Point:
The raw type (e.g., List) determines the signature, not the generic type (List<T>).
Comparison Table: Overloading vs. Overriding
Feature | Method Overloading | Method Overriding |
Signature | Name + different parameters | Name + same parameters |
Return Type | Can differ | Must match (or be covariant) |
Inheritance | Not required | Requires a subclass |
Polymorphism | Compile-time (static) | Runtime (dynamic) |
Access | No restriction on modifiers | Cannot reduce parent method’s access |
📌Pro Tip: Overloading = same class, Overriding = parent-child classes.
For authority, reference the official Java docs:
"A method signature consists of the method name and the number, type, and order of its formal parameters. The signature does not include the return type or thrown exceptions."
— Java Language Specification (§8.4.2)
Components of a Method Signature
- Method Name: The identifier used to invoke the method.
- Parameter List: Includes:
- Number of parameters.
- Data types of parameters.
- The order in which the parameters are defined.
Example:
void process(int a, double b) { }
void process(double b, int a) { } // Different signature
What Does Not Affect a Method Signature
- Return Type: Methods cannot be differentiated based on their return type alone.
int calculate() { return 0; }
void calculate() { } // Compilation error: duplicate method
- Access Modifiers: public, private, protected, or default do not change the signature.
- Throws Clause: Declaring exceptions with throws does not alter the method signature.
void readFile() throws IOException { }
void readFile() { } // Same signature, regardless of throws clause
Common Misconceptions
1. Return Type: The return type of a method is not part of its signature. For example:
void calculate();
int calculate(); // This will cause a compilation error
→ Despite having different return types, the above methods have identical signatures and cannot coexist.
2. Access Modifiers:
Modifiers like public, private, or protected do not influence the method signature. For example:
public void display();
private void display();
→ These methods have the same signature.
Summary
A method signature in java is a fundamental concept that forms the basis for features like method overloading, overriding, and polymorphism. By understanding it deeply, you can write clear and effective methods while avoiding common pitfalls.
Related Articles
Frequently Asked Questions
No, the throws clause specifying exceptions is not part of the method signature.
No, parameter names are irrelevant to the method signature. Only the types, count, and order of parameters matter.