When working with databases, you often need to retrieve data from multiple tables at once. This is where querying multiple tables via joins becomes essential. Joins allow you to combine rows from two or more tables based on a related column, making it easier to extract meaningful insights from your data.
In this blog, we’ll cover:
- Why joins are necessary
- Different types of joins (INNER JOIN, LEFT JOIN, RIGHT JOIN, FULL JOIN)
- How normalization affects joins
- Practical examples of querying multiple tables via joins
- Best practices for writing efficient join queries
By the end, you’ll have a solid understanding of how to use joins effectively in SQL.
Why Do We Need Joins?
Databases are designed to minimize redundancy through normalization, which splits data into multiple related tables. For example:
- A Books table stores book details.
- A Publishers table stores publisher information.
- An Authors table stores author details.
Instead of repeating the same publisher or author names in every book record, we use IDs to link tables. Querying multiple tables via joins allows us to:
- Combine these tables when retrieving data.
- Avoid duplicate data storage.
- Keep the database efficient and organized.
Understanding Database Normalization
Before diving into joins, it’s important to understand normalization, which structures databases to reduce redundancy. Know more about advanced normalization techniques like Boyce-Codd Normal Form (BCNF).
Example of a Denormalized Table
Consider a Books table with repeated publisher and author names:
Title | ISBN | Publish Date | Publisher | Author 1 | Author 2 | Author 3 |
Book 1 | 123456789 | June 1, 2008 | Publisher 1 | Author A | Author B |
|
Book 2 | 124356798 | Sept 12, 2009 | Publisher 2 | Author B | Author C |
|
Problems with this approach:
- The same publisher name repeats.
- Authors are stored in multiple columns.
- Updating a publisher name requires changing multiple rows.
Problems with this approach:
- The same publisher name repeats.
- Authors are stored in multiple columns.
- Updating a publisher name requires changing multiple rows.
Normalized Database Structure
To fix this, we split data into separate tables:
- Publisher Table
PublisherID | PublisherName |
1 | Publisher 1 |
2 | Publisher 2 |
- Author Table
AuthorID | AuthorName |
1 | Author A |
2 | Author B |
- Book Table (Now Simplified)
BookID | Title | ISBN | Publish Date | PublisherID |
1 | Book 1 | 123456789 | June 1, 2008 | 1 |
- BookAuthor (Bridge Table for Many-to-Many Relationship)
BookID | AuthorID |
1 | 1 |
1 | 2 |
Now, instead of repeating data, we use joins to connect tables when querying
Types of Joins in SQL
When querying multiple tables via joins, you’ll use four main types:
INNER JOIN (Default Join)
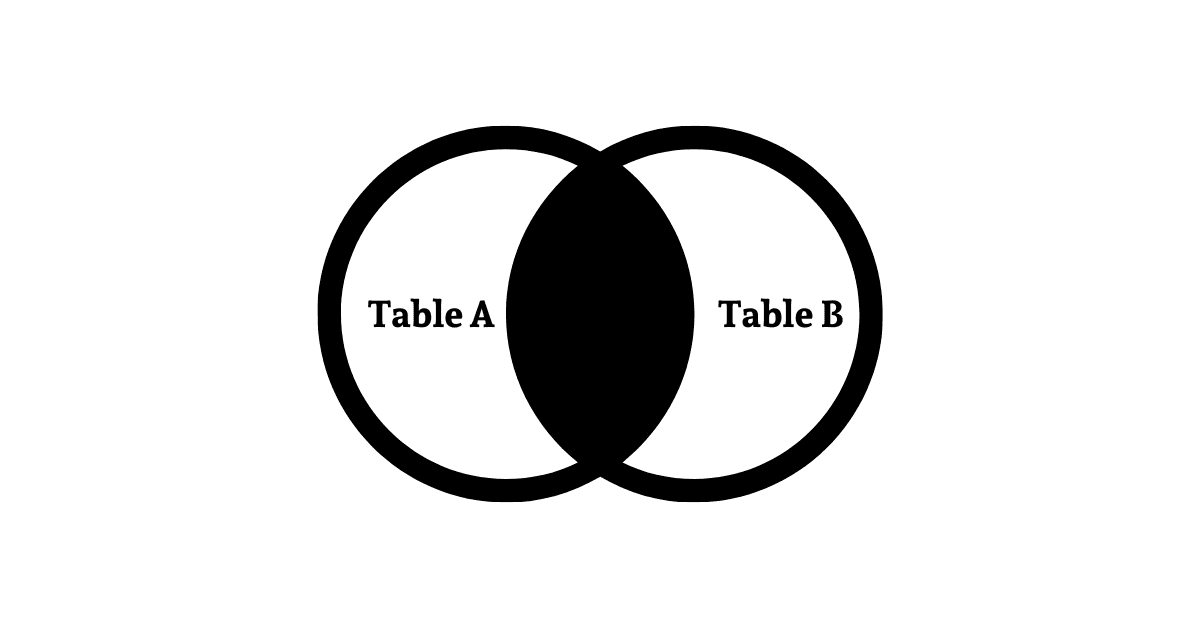
- Returns only matching rows from both tables.
- If no match exists, the row is excluded.
Example:
SELECT B.Title, P.PublisherName
FROM Book B
INNER JOIN Publisher P ON B.PublisherID = P.PublisherID
Result: Only books with a valid PublisherID appear.
LEFT JOIN (LEFT OUTER JOIN)
- Returns all rows from the left table (first table) and matching rows from the right table.
- If no match, NULL appears for right table columns.
Example:
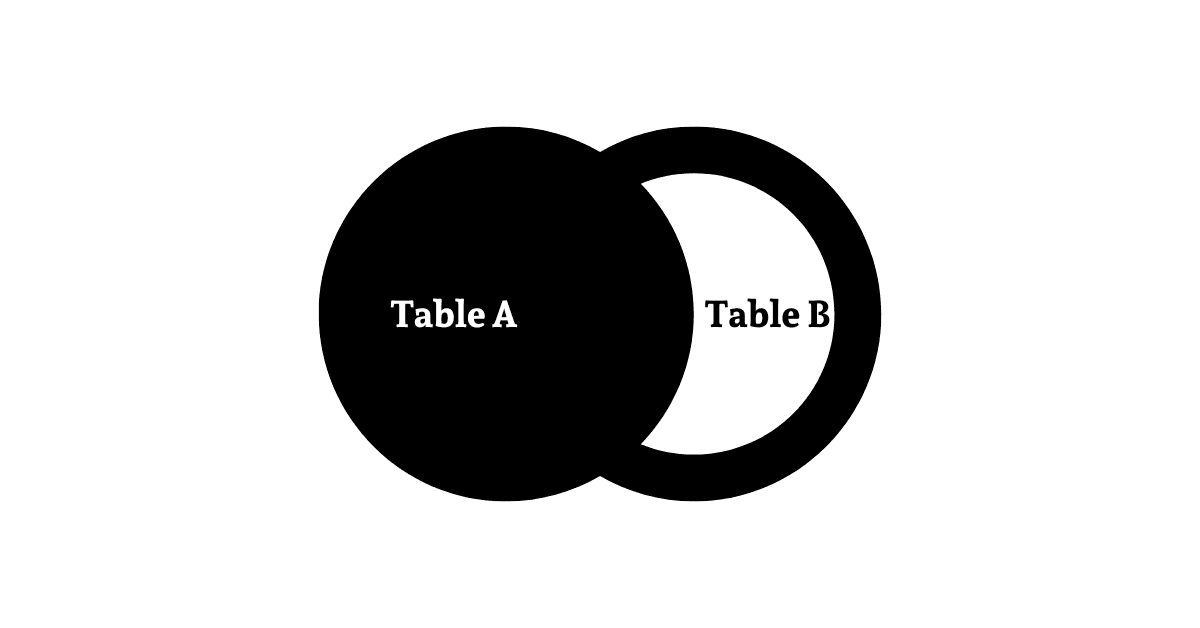
SELECT B.Title, P.PublisherName
FROM Book B
LEFT JOIN Publisher P ON B.PublisherID = P.PublisherID
Result: All books are listed, even if they have no publisher.
RIGHT JOIN (RIGHT OUTER JOIN)
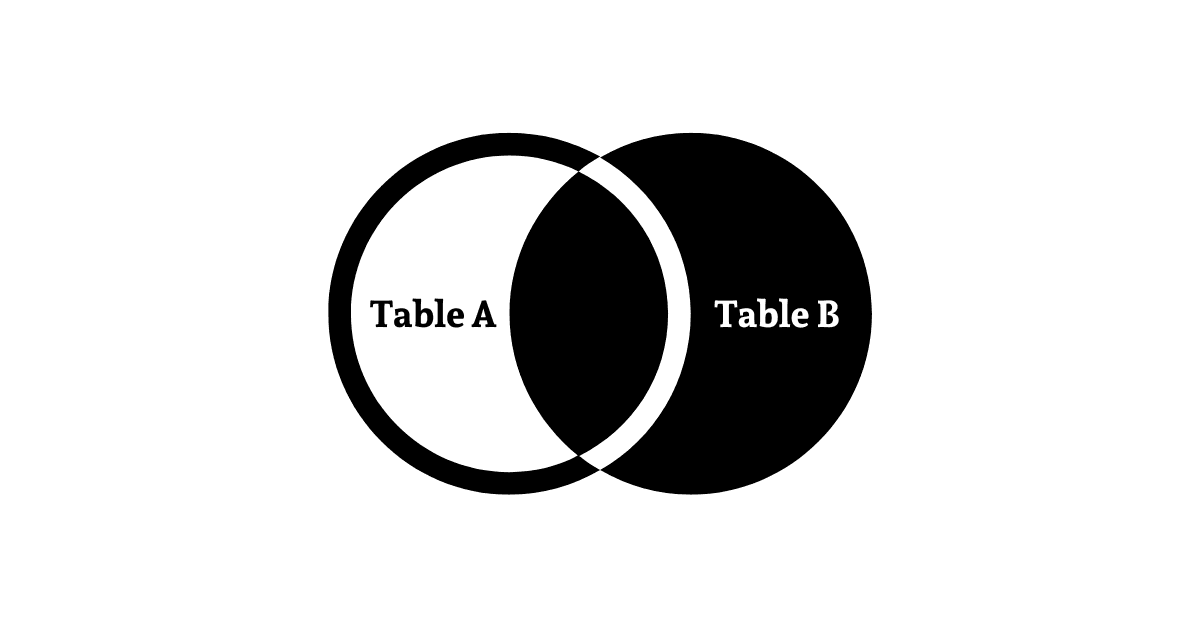
- Returns all rows from the right table and matching rows from the left table.
- If no match, NULL appears for left table columns.
Example:
SELECT B.Title, P.PublisherName
FROM Book B
RIGHT JOIN Publisher P ON B.PublisherID = P.PublisherID
Result: All publishers are listed, even if they have no books.
FULL JOIN (FULL OUTER JOIN)
- Returns all rows from both tables.
- If no match, NULL fills the missing side.
Example:
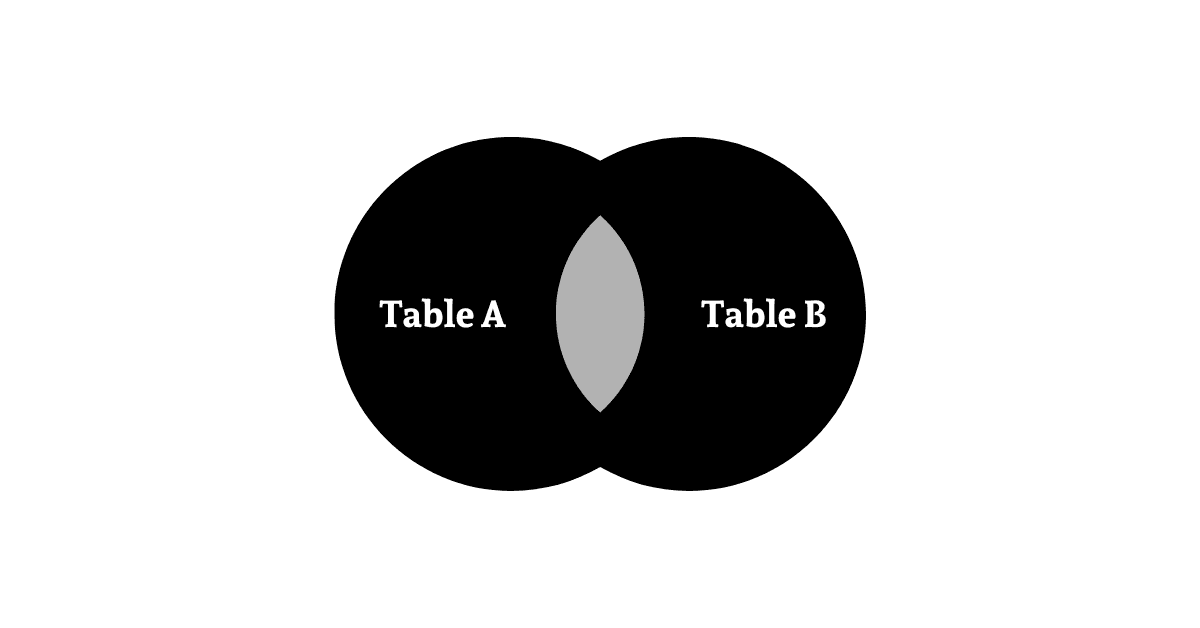
SELECT B.Title, P.PublisherName
FROM Book B
FULL JOIN Publisher P ON B.PublisherID = P.PublisherID
Result: Combines all books and all publishers, with NULL where no match exists.
SQL Joins Cheat Sheet📋
(Save this for quick reference!)
Practical Examples of Querying Multiple Tables via Joins
Example 1: Books and Publishers (One-to-Many Relationship)
SELECT B.Title, B.ISBN, P.PublisherName
FROM Book B
INNER JOIN Publisher P ON B.PublisherID = P.PublisherID
Output:
Title | ISBN | PublisherName |
Book 1 | 123456789 | Publisher 1 |
Example 2: Books and Authors (Many-to-Many Relationship)
SELECT B.Title, A.AuthorName
FROM Book B
INNER JOIN BookAuthor BA ON B.BookID = BA.BookID
INNER JOIN Author A ON BA.AuthorID = A.AuthorID
Output:
Title | AuthorName |
Book 1 | Author A |
Book 1 | Author B |
Example 3: LEFT JOIN to Include Missing Data
SELECT B.Title, P.PublisherName
FROM Book B
LEFT JOIN Publisher P ON B.PublisherID = P.PublisherID
Output:
Title | PublisherName |
---|---|
Book 1 | Publisher 1 |
Book X | NULL |
NULL → (if no publisher exists)
Best Practices for Querying Multiple Tables via Joins
- Use Table Aliases – Improves readability.
SELECT B.Title, P.PublisherName FROM Book B INNER JOIN Publisher P ON B.PublisherID = P.PublisherID
- Choose the Right Join Type
- Use INNER JOIN for strict matches.
- Use LEFT JOIN to keep all records from the first table.
- Avoid Too Many Joins – Can slow down queries.
- Index Foreign Keys – Speeds up join operations.
- Test with Small Data First – Ensure joins work before running on large tables.
- Index foreign keys - Speeds up join operations by 10x+ in large tables
- Use EXPLAIN - Reveals if joins are using indexes properly
- Limit joined columns - Only select what you need (SELECT a.id, b.name vs SELECT *)
- Filter early - Apply WHERE clauses before joining when possible
Advanced Join Optimization Techniques
1. Join Performance Tuning
🔧 Problem: Your 5-table join query runs slowly.
✅ Solution:
→ Index Strategically
CREATE INDEX idx_author_book ON BookAuthor(BookID, AuthorID);
- Use Query Hints (SQL Server example):
SELECT * FROM Book WITH (NOLOCK) INNER JOIN Publisher...
- Limit Joined Data Early:
SELECT * FROM (
SELECT id, name FROM Customers WHERE region='West'
) c JOIN Orders o ON c.id = o.customer_id
📌Pro Tip: Learn how database architecture affects performance with Three-Schema Architecture in DBMS.
2. Hash Joins vs. Nested Loops
Join Type | Best For | Example Use Case |
Hash Join | Large tables | Data warehousing |
Nested Loop | Small-to-medium | OLTP with indexed columns |
Conclusion
Querying multiple tables via joins is a fundamental skill in SQL. Whether you’re using INNER JOIN, LEFT JOIN, or other types, joins help you combine data efficiently.
Key Takeaways:
- Normalization reduces redundancy but requires joins to retrieve related data.
- INNER JOIN returns only matching rows.
- LEFT JOIN keeps all left table records, even without matches.
- Many-to-many relationships need a bridge table.
By mastering joins, you’ll be able to write powerful SQL queries that pull data from multiple sources seamlessly.
Now it’s your turn! Try writing some join queries on your database and see how they work. Happy querying! 🚀
1 thought on “Querying Multiple Tables via Joins: A Complete Guide”
Comments are closed.